속성 값 설정
타임리프 태그 속성(Attribute)
타임리프는 주로 HTML 태그에 th:* 속성을 지정하는 방식으로 동작한다.
th:* 로 속성을 적용하면 기존 속성을 대체한다. 기존 속성이 없으면 새로 만든다.
<input type="text" name="hi" th:name="test" />
- input 태그의 name속성의 값을 "hi"로 설정한 후 th:name ="test"라는 타임리프의 속성을 지정했다면
최종 결과 값은 다음과 같이 렌더링 된다.
<input type="text" name="test" />
속성 추가
속성의 이름을 변경하는 것 말고도 속성을 추가하는 일도 빈번하게 발생한다.
타임리프는 th:attrappend로 속성을 추가할 수 있다.
<input type="text" class="text" th:attrappend="class=' large'" />
여기서 주의할 점은 타임리프의 attrappend="class=' large'" 작은 따옴표를 사용했다는 점이다. 또한 한 칸 띄웠다는 점이다.
<input type="text" class="text large"/>
th:attrappend는 뒤에 붙지만 앞에 넣어야 할 경우에는 th:attrprepend로 사용하고 뒤에 빈칸을 한 칸 띄워주면 된다.
<input type="text" class="text" th:attrprepend="class='large '" />
빈칸을 신경 쓰기 귀찮다면 다음과 같이 제공해주는 th:classappend를 사용하면 된다.
<input type="text" class="text" th:classappend="large" />
자주 사용되는 input 태그의 checkbox 속성에는 간과할 수 있는 문제가 하나 있다.
https://stackoverflow.com/questions/4228658/what-values-for-checked-and-selected-are-false
<input type="checkbox" checked />
<input type="checkbox" checked="" />
<input type="checkbox" checked="checked" />
<input type="checkbox" checked="unchecked" />
<input type="checkbox" checked="true" />
<input type="checkbox" checked="false" />
<input type="checkbox" checked="on" />
<input type="checkbox" checked="off" />
<input type="checkbox" checked="1" />
<input type="checkbox" checked="0" />
<input type="checkbox" checked="yes" />
<input type="checkbox" checked="no" />
<input type="checkbox" checked="y" />
<input type="checkbox" checked="n" />
어떠한 값이 들어가도 checked라는 속성 이름만 있으면 모조리 체크되는 것이 HTML5 사양이다.
<h1>checked 처리</h1>
- checked o <input type="checkbox" name="active" th:checked="true" /><br/>
- checked x <input type="checkbox" name="active" th:checked="false" /><br/>
- checked=false <input type="checkbox" name="active" checked="false" /><br/>
타임리프에서는 th:checked라는 속성을 제공한다.
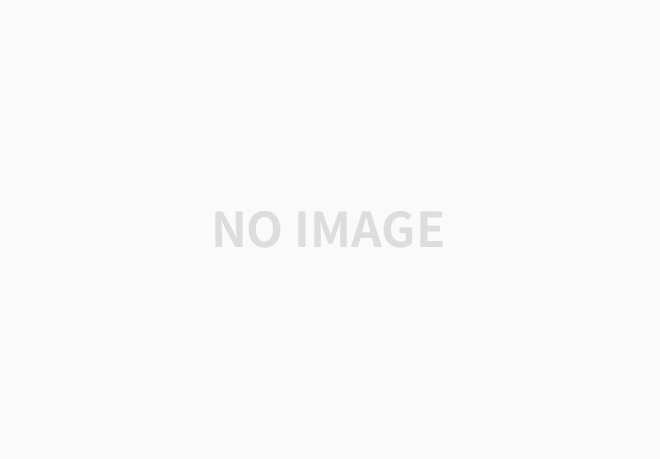
반복
타임리프에서 반복은 th:each를 사용한다. 추가로 반복에서 사용할 수 있는 여러 상태 값을 지원한다.
<table border="1">
<tr>
<th>username</th>
<th>age</th>
</tr>
<tr th:each="user : ${users}">
<td th:text="${user.username}">username</td>
<td th:text="${user.age}">0</td>
</tr>
</table>
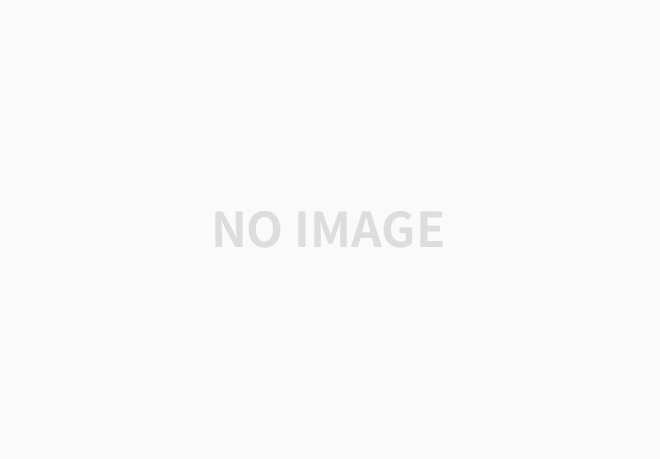
기본 반복 기능
- 오른쪽에 반복 가능한 요소 java.util, Iterable, java.util.Enumeration을 구현한 모든 객체를 반복에서 사용할 수 있으며 java.util.Map을 구현한 객체인 경우 entry를 반환하여 getValue(), getKey()로 조회할 수 있습니다.
반복 상태 유지
반복의 상태라는 것은 index, 반복 횟수, 크기, 짝수, 홀수, 현재 등등 상태에 접근할 수 있는 방법을 제공합니다.
<tr th:each="user, userStat : ${users}">
<td th:text="${userStat.count}">username</td>
<td th:text="${user.username}">username</td>
<td th:text="${user.age}">0</td>
<td>
index = <span th:text="${userStat.index}"></span>
count = <span th:text="${userStat.count}"></span>
size = <span th:text="${userStat.size}"></span>
even? = <span th:text="${userStat.even}"></span>
odd? = <span th:text="${userStat.odd}"></span>
first? = <span th:text="${userStat.first}"></span>
last? = <span th:text="${userStat.last}"></span>
current = <span th:text="${userStat.current}"></span>
</td>
</tr>
두 번째 파라미터를 설정해서 반복의 상태를 확인할 수 있습니다.
두 번째 파라미터는 생략 가능한데, 생략하면 지정한 변수 (user) + Stat으로 접근할 수 있습니다.
* current : 현재 객체를 의미합니다.
타임리프의 조건식 if , unless ( if의 반대 )
- 조건을 만족해야 해당 태그를 출력합니다.
<span th:text="${user.age}">0</span>
<span th:text="'미성년자'" th:if="${user.age lt 20}"></span>
<span th:text="'미성년자'" th:unless="${user.age ge 20}"></span>
switch문도 사용할 수 있습니다.
<div th:switch="${user.age}>
<span th:case="10">10</span>
<span th:case="20">20</span>
<span th:case="*">else</span>
</div>
블록
HTML 태그가 아닌 타임리프의 유일한 자체 태그인 <th:block>가 존재한다.
앵귤러를 접 한 사람은 쉽게 이해할 수 있는 ng-container와 같은 역할을 한다.
여러 개의 묶음을 반복을 하는데, 테두리 태그가 존재하지 않아야 하는 경우 매우 유용하게 사용할 수 있다.
<th:block th:each="user : ${users}">
<div>
사용자 이름1 <span th:text="${user.username}"></span>
사용자 나이1 <span th:text="${user.age}"></span>
</div>
<div>
요약 <span th:text="${user.username} + ' / ' + ${user.age}"></span>
</div>
</th:block>
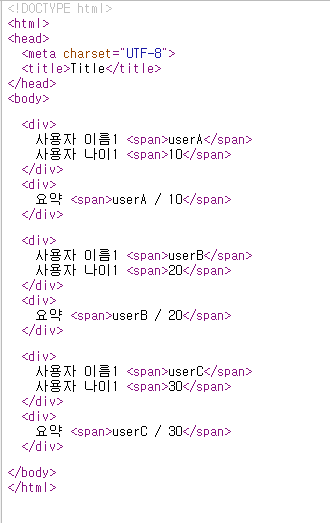
결과를 확인해보면 반복되는 두 개의 div태그들이 컬렉션의 size만큼 반복되는 것을 확인할 수 있다.
'SSR > Thymeleaf' 카테고리의 다른 글
Thymeleaf (5) (0) | 2022.01.05 |
---|---|
Thymeleaf (4) (0) | 2022.01.05 |
Thymeleaf (2) (0) | 2022.01.04 |
Thymeleaf (1) (0) | 2022.01.03 |
댓글